The Event Loop: Why JavaScript Isn’t Really Single-Threaded
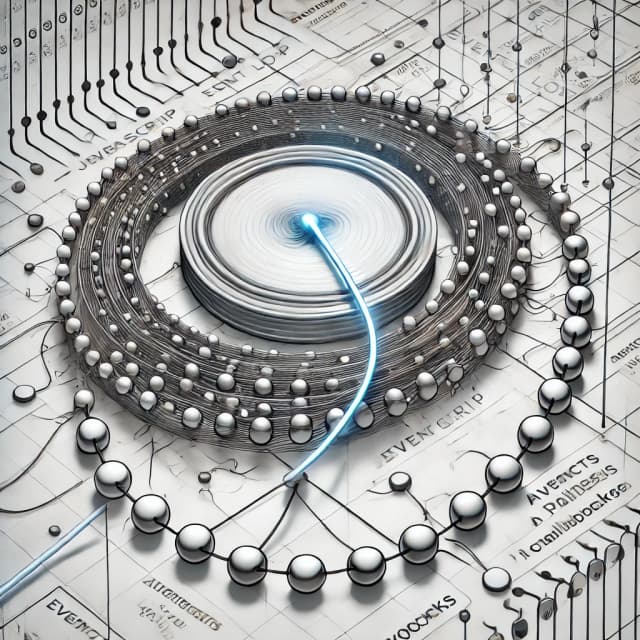
The Event Loop: Why JavaScript Isn’t Really Single-Threaded
JavaScript is often said to be a single-threaded language. But what does that even mean? And if JavaScript is single-threaded, how can it handle things like asynchronous tasks, such as waiting for data from a server? How does it manage all of this without blocking the rest of the code? The answer is the Event Loop.
Let’s dive in and break it down! 🚀
🤔 What Does Single-Threaded Mean?
When we say JavaScript is single-threaded, we mean that it runs on just one thread of execution at a time. A thread is essentially a sequence of instructions that the CPU follows to perform tasks.
If you’ve worked with other programming languages, you might know that they can be multi-threaded, meaning they can execute multiple pieces of code at once. But JavaScript, by default, only runs one thing at a time.
So, how does JavaScript manage multiple tasks like HTTP requests, timers, or animations without freezing up? The secret is in the Event Loop.
🔄 The Event Loop: JavaScript’s Magic Behind the Scenes
At the heart of how JavaScript handles multiple tasks is the Event Loop. The Event Loop is a mechanism that allows JavaScript to perform non-blocking asynchronous operations, even though it's single-threaded. Here’s how it works:
Call Stack: This is where the functions that are currently executing live. The browser or Node.js processes one function at a time here.
Web APIs: When JavaScript encounters things like
setTimeout()
,fetch()
, or event listeners, these tasks are handled by the browser’s Web APIs, not the JavaScript engine directly. These tasks run in the background, outside the call stack.Callback Queue: Once the Web APIs finish their job (like fetching data or waiting for a timeout), they send their results to the callback queue. This queue holds the functions that need to be executed once the call stack is empty.
Event Loop: The Event Loop constantly checks if the call stack is empty. If it is, it pushes the first function from the callback queue to the call stack for execution.
🔍 Visualizing the Event Loop
Let’s look at an example to see how the Event Loop works.
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 2000);
console.log('End');
🤯 What Happens Here?
'Start'
is logged immediately because it's on the call stack.setTimeout()
is called, but the callback function is handed off to the Web API to wait for 2 seconds.'End'
is logged immediately after because it's also on the call stack.- After 2 seconds, the callback function from
setTimeout()
moves to the callback queue. - The Event Loop sees that the call stack is empty, so it moves the callback function from the queue to the stack, where
'Timeout'
is logged.
🕒 Non-Blocking & Asynchronous Operations
The key takeaway here is that JavaScript is non-blocking. While JavaScript is waiting for something (like data from a server or a timer), it doesn't freeze or block other tasks. It’s able to run other code while waiting, thanks to the Event Loop.
For example, the code above doesn’t wait for the timeout to finish before logging 'End'
. It doesn't block the execution of 'End'
, which demonstrates the asynchronous nature of JavaScript.
🧑💻 Why Does This Matter?
Knowing how the Event Loop works can help you write more efficient code, especially in environments like web browsers and Node.js where asynchronous operations are crucial. It’s also important for understanding concepts like:
- Concurrency: Managing multiple operations at once without blocking the main thread.
- Callbacks: Functions passed as arguments to be executed later.
- Promises: A cleaner way to handle asynchronous operations.
🚀 Final Thoughts
JavaScript is indeed single-threaded, but it’s far from being limited. With the Event Loop, JavaScript can handle multiple tasks at once by processing asynchronous events without blocking the main thread. Understanding how the Event Loop works opens up a whole new level of understanding for JavaScript, helping you write better and more efficient code.
Got any questions about the Event Loop or asynchronous programming? Let’s talk in the comments! 🚀