Closures Explained Like You’re Five
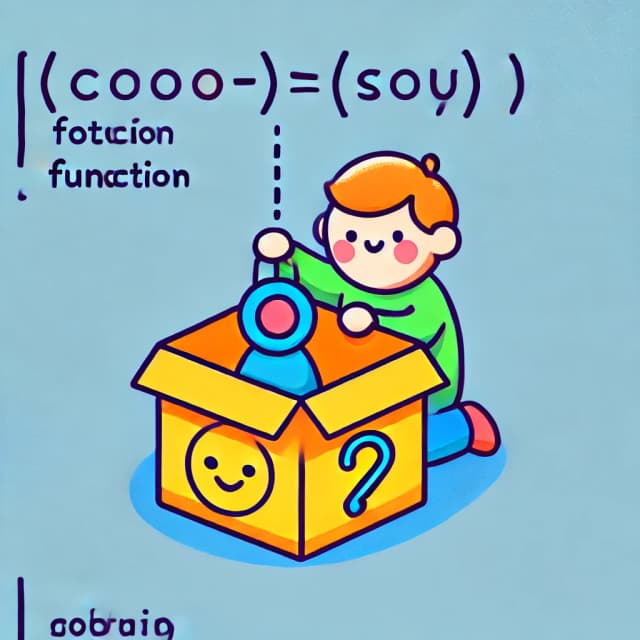
Closures Explained Like You’re Five
Closures might sound like a complicated concept, but they are actually pretty simple once you break them down. If you've ever heard the term and thought, "What is that?"—don’t worry! Let’s explain closures in a way that even a five-year-old could understand.
🎭 Imagine a Magic Backpack
Imagine you have a magic backpack. Anything you put inside this backpack stays with you forever, even if you leave the room where you packed it. You can take things out whenever you want, but no one else can see what's inside.
Closures in JavaScript work just like that! When a function is created inside another function, it remembers the variables from the outer function—even after the outer function has finished running.
Let’s see it in action! 🎬
🏗 A Simple Closure Example
function outerFunction() {
let name = "Alice"; // This variable is inside the outer function
function innerFunction() {
console.log("Hello, " + name); // The inner function remembers "name"
}
return innerFunction;
}
const myClosure = outerFunction(); // The outer function runs and returns innerFunction
myClosure(); // "Hello, Alice" (innerFunction still remembers "name")
🔍 What’s Happening Here?
outerFunction
creates a variablename
.- Inside
outerFunction
, there’s another function calledinnerFunction
. innerFunction
usesname
, but it’s insideouterFunction
.- When we run
outerFunction()
, it returnsinnerFunction
, but the magic happens wheninnerFunction
still remembersname
, even afterouterFunction
has finished running!
This is a closure—a function that remembers the variables from where it was created.
🎮 Another Example: Making a Counter
Closures are useful for keeping data private and creating things like counters.
function createCounter() {
let count = 0;
return function () {
count++;
console.log("Current count: " + count);
};
}
const counter = createCounter();
counter(); // "Current count: 1"
counter(); // "Current count: 2"
counter(); // "Current count: 3"
🤯 Why Does This Work?
count
is insidecreateCounter
, so it’s not directly accessible from outside.- The returned function remembers
count
because of closures. - Every time we call
counter()
, it increases the storedcount
.
Without closures, count
would be reset every time createCounter()
is called.
🚀 Why Should You Care?
Closures are everywhere in JavaScript. They are useful for:
- Encapsulating private variables (like in the counter example).
- Handling asynchronous operations (like in
setTimeout
). - Event listeners and callbacks.
- Creating factory functions and modules.
🏁 Final Thoughts
If closures still seem tricky, don’t worry! The best way to understand them is to experiment. Try writing your own closure-based functions and see how they remember variables.
Remember the magic backpack analogy: A closure holds onto variables even after the outer function is gone.
Got any questions or examples where closures confused you? Let’s discuss in the comments! 🚀